Demo
Demo of the React Native QRCode Pack @masumdev/rn-qrcode and @masumdev/rn-qrcode-scanner component on Android and iOS devices.
QRCode @masumdev/rn-qrcode
QRCode Scanner @masumdev/rn-qrcode-scanner
Please note that the QR Code Scanner (@masumdev/rn-qrcode-scanner) is currently only supported on Android devices due to compatibility issues with Expo's camera implementation on iOS. We are actively working on a solution and iOS support will be available in future releases.
Overview
QRCode Overview
Experience our powerful QR Code generator in action across both Android and iOS platforms. The QR Code component offers extensive customization options, high performance, and seamless integration with your React Native applications.
import { QRCode } from '@masumdev/rn-qrcode';
function QRCodeExample() {
return (
<QRCode
value="https://example.com"
size={200}
logo={require('./assets/logo.png')}
gradientColors={['#FF6B6B', '#4ECDC4']}
errorCorrectionLevel="H"
/>
);
}
Android Demo
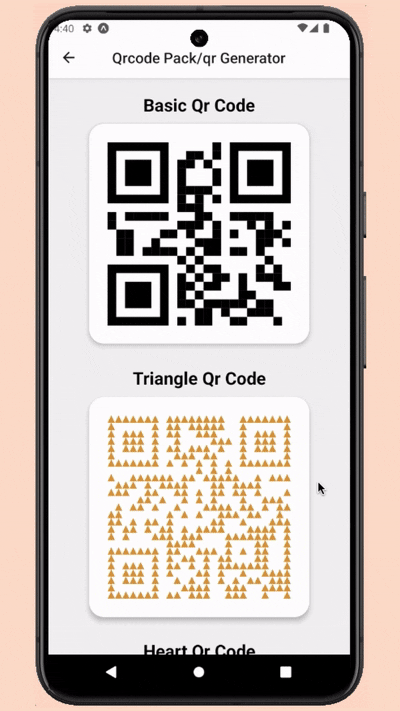
iOS Demo
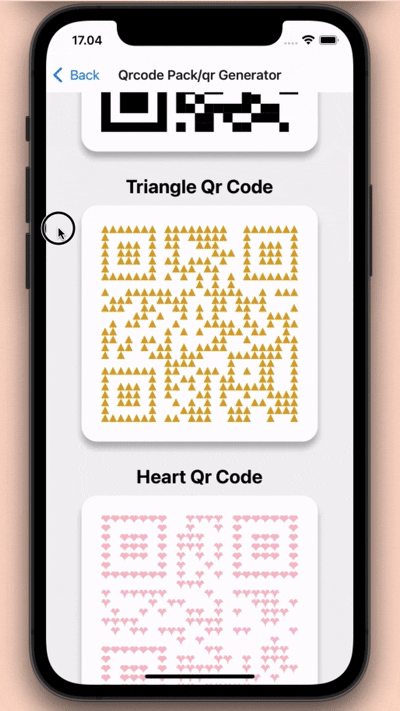
QRCode Scanner Overview
The QR Code Scanner component provides a robust, easy-to-implement solution for scanning QR codes in your React Native application. It features real-time scanning, customizable UI, and comprehensive format support.
import { QRCodeScanner } from '@masumdev/rn-qrcode-scanner';
function ScannerExample() {
const handleScan = (result) => {
console.log('Scanned:', result);
};
return (
<QRCodeScanner
onScan={handleScan}
vibrate={true}
flashEnabled={false}
scanInterval={2000}
style={{
container: { flex: 1 },
marker: { borderColor: '#2ecc71' }
}}
/>
);
}
Android Demo
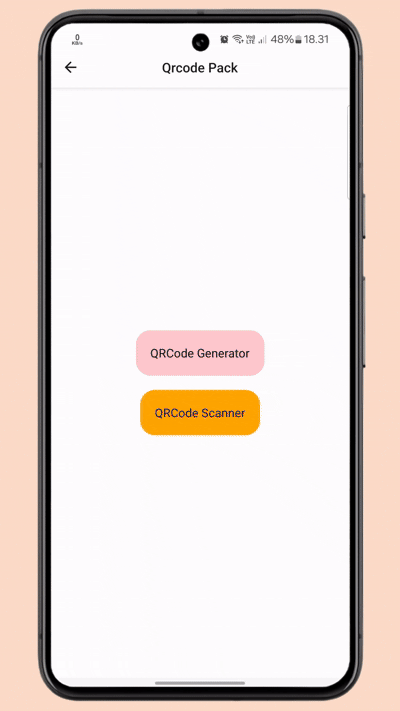
iOS Demo
Unsupported on iOS at the momentFeatures
QRCode Features
- 🎨 Rich QR Code styling with fully customizable:
- Colors and gradients
- Patterns and shapes
- Corner styles and dot designs
- Background effects
- 🖼️ Custom logo placement with adjustable size and position
- 🌈 Beautiful preset templates and themes
- 🔍 Comprehensive format support for:
- URLs and deep links
- Contact information (vCard, meCard)
- Calendar events
- Wi-Fi configurations
- And more!
- 🎯 Adjustable error correction levels (L, M, Q, H)
- 📦 Seamless integration with React Native projects
- 💫 Cross-platform compatibility (iOS & Android)
- ⚡ High-performance QR code generation
- 🎭 Multiple design variants and styles
QRCode Scanner Features
- 📱 Advanced QR Code scanning with camera support
- 🔍 Multiple format support (URL, text, contact info, etc.)
- 💫 Real-time scanning with high performance
- 🎯 Customizable scanning area and frame
- 🛡️ Built-in camera permission handling
- 📳 Haptic feedback on successful scans
- 🎨 Customizable UI elements (corners, overlay, controls)
- 🔦 Torch/flashlight control support
- ⏱️ Cooldown timer between scans
- ✅ Custom validation support
- 🌓 Theme support (light/dark mode)
- ⚡ Optimized for both iOS and Android
Key Interactions Demonstrated
QR Code Generation
- Dynamic QR code creation with real-time updates
- Customizable styling and appearance
- Logo integration with automatic positioning
- Multiple format encoding support
- Error correction level handling
Scanner Interactions
- Real-time camera feed processing
- Multi-format QR code detection
- Haptic feedback on successful scans
- Torch/flashlight control
- Permission handling flow
Customization Features
- Rich styling options for QR codes
- Gradient and pattern customization
- Scanner UI theming support
- Frame and overlay customization
- Responsive layout adaptation
To achieve optimal performance:
- Choose appropriate error correction levels
- Optimize logo size and placement
- Handle camera permissions properly
- Implement proper error handling
- Consider scan area optimization
Watch out for these issues:
- Large logos affecting QR code readability
- Excessive styling affecting scan performance
- Improper camera resource management
- Memory leaks from unmanaged listeners
- Heavy UI animations during scanning
Try It Yourself
To get started with this component in your own project, check out our installation guide and basic usage examples.